Screen Shot:
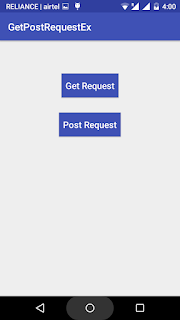
Download Source Code
Step 1:
Open activity_main.xml file and copy the below code.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.prasad.getpostrequestex.MainActivity"> <Button android:id="@+id/getRequest" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_marginTop="50dp" android:background="@color/colorPrimary" android:padding="8dp" android:text="Get Request" android:textAllCaps="false" android:textColor="#ffffff" android:textSize="18dp" /> <Button android:id="@+id/postRequest" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/getRequest" android:layout_centerHorizontal="true" android:layout_margin="30dp" android:background="@color/colorPrimary" android:padding="8dp" android:text="Post Request" android:textAllCaps="false" android:textColor="#ffffff" android:textSize="18dp" /> </RelativeLayout>
Step 2:
Create ConnectionDetector.java class to check Internet connection and copy below code.
package com.prasad.getpostrequestex.background; import android.content.Context; import android.net.ConnectivityManager; import android.net.NetworkInfo; public class ConnectionDetector { /** * Checking for all possible internet providers * **/ public static boolean isConnectingToInternet(Context _context){ ConnectivityManager connectivity = (ConnectivityManager) _context.getSystemService(Context.CONNECTIVITY_SERVICE); if (connectivity != null) { NetworkInfo[] info = connectivity.getAllNetworkInfo(); if (info != null) for (int i = 0; i < info.length; i++) if (info[i].getState() == NetworkInfo.State.CONNECTED) { return true; } } return false; } }
Step 3:
Create ServiceResponse.java model class to store success or failure response and copy below code.
package com.prasad.getpostrequestex.background; public class ServiceResponse { private boolean isSuccessOrFail; private String jsonResponse; public void setIsSuccessOrFail(boolean isSuccessOrFail) { this.isSuccessOrFail = isSuccessOrFail; } public boolean isSuccessOrFail() { return isSuccessOrFail; } public String getJsonResponse() { return jsonResponse; } public void setJsonResponse(String jsonResponse) { this.jsonResponse = jsonResponse; } public ServiceResponse(boolean isSuccessOrFail, String jsonResponse) { this.isSuccessOrFail = isSuccessOrFail; this.jsonResponse = jsonResponse; } }
Step 4:
Create WebServiceCallBack.java interface to return response to activity class and copy below code.
package com.prasad.getpostrequestex.background; public interface WebServiceCallBack { void onResponseReceived(Object response); }
Step 5:
Create WebServiceAsyncTask.java class to call background assync task and copy below code.
package com.prasad.getpostrequestex.background; import android.app.ProgressDialog; import android.content.Context; import android.os.AsyncTask; import java.util.HashMap; public class WebServiceAsyncTask extends AsyncTask<Object, Void, Object> { private ProgressDialog pDialog; private Context context; private WebServiceCallBack webServiceListener; private String requestUrl; private HashMap<String, String> extraParams; private int requestType; private boolean showProgressBar; public WebServiceAsyncTask(Context context, WebServiceCallBack webServiceListener, String requestUrl, HashMap<String, String> extraParams, int requestType, boolean showProgressBar) { this.context = context; this.webServiceListener = webServiceListener; this.requestUrl = requestUrl; this.extraParams = extraParams; this.requestType = requestType; this.showProgressBar = showProgressBar; } @Override protected void onPreExecute() { super.onPreExecute(); if (showProgressBar) { showProgressDialog(); } } @Override protected Object doInBackground(Object[] params) { synchronized (this) { boolean networkStatus = ConnectionDetector.isConnectingToInternet(context); ServiceResponse serviceResponse = null; if (networkStatus) { HttpWebServiceHandler serviceHandler = new HttpWebServiceHandler(); if (requestType == 1) { serviceResponse = serviceHandler.getServiceCall(requestUrl, extraParams); } else if (requestType == 2) { serviceResponse = serviceHandler.postServiceCall(requestUrl, extraParams); } } else { serviceResponse = new ServiceResponse(false, HttpWebServiceHandler.NoNetWorkErrorMSG); } return serviceResponse; } } @Override protected void onPostExecute(Object reponse) { super.onPostExecute(reponse); try { webServiceListener.onResponseReceived(reponse); if (showProgressBar) { dismissProgressDialog(); showProgressBar = false; } } catch (final IllegalArgumentException e) { } catch (final Exception e) { } finally { pDialog = null; } } public void showProgressDialog() { pDialog = new ProgressDialog(context); pDialog.setMessage("Please wait..."); pDialog.setCancelable(false); if (!pDialog.isShowing()) pDialog.show(); } public void dismissProgressDialog() { if (pDialog != null && pDialog.isShowing()) pDialog.dismiss(); } }
Step 6:
Create HttpWabServiceHandler.java class file to call Get and Post requests and copy below code.
package com.prasad.getpostrequestex.background; import android.util.Log; import org.json.JSONObject; import java.io.BufferedInputStream; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.io.OutputStream; import java.io.OutputStreamWriter; import java.io.UnsupportedEncodingException; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.util.HashMap; import java.util.Map; import javax.net.ssl.HttpsURLConnection; public class HttpWebServiceHandler { static String response = null; public static final String NoNetWorkErrorMSG = "No Internet Connection !!!"; public static final String ServerErrorMSG = "Error in Connection !!!"; private static JSONObject jsonObj; public HttpWebServiceHandler() { } // Get Request using HttpURLConnection Code public ServiceResponse getServiceCall(String requestURL, HashMap<String, String> params) { InputStream inputStream = null; HttpURLConnection conn = null; ServiceResponse serviceResponse = null; String newRequestURL = ""; try { if (params != null) { newRequestURL = requestURL + getQueryString(params); } else { newRequestURL = requestURL; } URL url = new URL(newRequestURL); Log.i("URL ", newRequestURL); conn = (HttpURLConnection) url.openConnection(); /* optional request header */ conn.setRequestProperty("Content-Type", "application/json"); /* optional request header */ conn.setRequestProperty("Accept", "application/json"); conn.setReadTimeout(500000); conn.setConnectTimeout(500000); /* for Get request */ conn.setRequestMethod("GET"); int statusCode = conn.getResponseCode(); /* 200 represents HTTP OK */ if (statusCode == HttpsURLConnection.HTTP_OK) { inputStream = new BufferedInputStream(conn.getInputStream()); response = convertInputStreamToString(inputStream); Log.i("Response", response); serviceResponse = new ServiceResponse(true, response); } else { response = ServerErrorMSG; serviceResponse = new ServiceResponse(false, response); } } catch (Exception e) { e.printStackTrace(); response = ServerErrorMSG; serviceResponse = new ServiceResponse(false, response); } finally { if (conn != null) conn.disconnect(); } // Log.i("RESPONSE", response); return serviceResponse; // return response } // POST Request using HttpURLConnection Code public ServiceResponse postServiceCall(String requestURL, HashMap<String, String> postDataParams) { InputStream inputStream = null; URL url; ServiceResponse serviceResponse = null; HttpURLConnection conn = null; try { String postParams = getQueryString(postDataParams); url = new URL(requestURL); conn = (HttpURLConnection) url.openConnection(); conn.setReadTimeout(500000); conn.setConnectTimeout(500000); conn.setRequestMethod("POST"); conn.setDoInput(true); conn.setDoOutput(true); conn.setRequestProperty("Content-Type", "application/x-www-form-urlencoded"); OutputStream os = conn.getOutputStream(); BufferedWriter writer = new BufferedWriter( new OutputStreamWriter(os, "UTF-8")); writer.write(postParams); writer.flush(); writer.close(); os.close(); int responseCode = conn.getResponseCode(); if (responseCode == HttpsURLConnection.HTTP_OK) { inputStream = new BufferedInputStream(conn.getInputStream()); response = convertInputStreamToString(inputStream); serviceResponse = new ServiceResponse(true, response); Log.i("Response", response); } else { response = ServerErrorMSG; serviceResponse = new ServiceResponse(false, response); } } catch (Exception e) { e.printStackTrace(); response = ServerErrorMSG; serviceResponse = new ServiceResponse(false, response); } finally { if (conn != null) conn.disconnect(); } return serviceResponse; } private String convertInputStreamToString(InputStream inputStream) throws IOException { BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(inputStream)); String line = ""; String result = ""; while ((line = bufferedReader.readLine()) != null) { result += line; } /* Close Stream */ if (null != inputStream) { inputStream.close(); } return result; } private String getQueryString(HashMap<String, String> params) throws UnsupportedEncodingException { StringBuilder result = new StringBuilder(); boolean first = true; for (Map.Entry<String, String> entry : params.entrySet()) { if (first) first = false; else result.append("&"); result.append(URLEncoder.encode(entry.getKey(), "UTF-8")); result.append("="); result.append(URLEncoder.encode(entry.getValue(), "UTF-8")); } return result.toString(); } }
Step 7:
Open MainActivity.java class file and copy below code.
package com.prasad.getpostrequestex; import android.content.Context; import android.content.DialogInterface; import android.os.Bundle; import android.support.v7.app.AlertDialog; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import com.prasad.getpostrequestex.background.ServiceResponse; import com.prasad.getpostrequestex.background.WebServiceAsyncTask; import com.prasad.getpostrequestex.background.WebServiceCallBack; import java.util.HashMap; public class MainActivity extends AppCompatActivity implements WebServiceCallBack { private Button getRequestBtn, postRequestBtn; private int serviceRequest = 1; private String url; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initControls(); } private void initControls() { getRequestBtn = (Button) findViewById(R.id.getRequest); postRequestBtn = (Button) findViewById(R.id.postRequest); getRequestBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { getRequestCall(); } }); postRequestBtn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { postRequestCall(); } }); } private void getRequestCall() { serviceRequest = 1; url = "http://androidexample.com/media/webservice/JsonReturn.php"; //Replace with your url WebServiceAsyncTask loginAsyncTask = new WebServiceAsyncTask(this, this, url, null, 1, true); loginAsyncTask.execute(); } private void postRequestCall() { serviceRequest = 2; url = ""; //Replace with your url // if you want pass parameters,use hash map like below HashMap<String, String> params = new HashMap<String, String>(); // params.put("userName", userName); // params.put("password", password); WebServiceAsyncTask loginAsyncTask = new WebServiceAsyncTask(this, this, url, params, 2, true); loginAsyncTask.execute(); } @Override public void onResponseReceived(Object response) { try { ServiceResponse serviceResponse = (ServiceResponse) response; String jsonResponse = serviceResponse.getJsonResponse(); if (serviceResponse.isSuccessOrFail()) { //Get Request response if (serviceRequest == 1) { //As per response parse json object } else if (serviceRequest == 2) { //Post Request response //As per response parse json object } } else { showAlertDialog(this, jsonResponse); } } catch (NullPointerException e) { e.printStackTrace(); } } public static void showAlertDialog(Context context, String message) { new AlertDialog.Builder(context) .setTitle(context.getResources().getString(R.string.app_name)) .setCancelable(false) .setMessage(message) .setPositiveButton("OK", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { } }) .show(); } }
Step 8:
Open AndroidManifest.xml file and add the internet permissions.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.prasad.getpostrequestex"> <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>